Hiding Scrollbars in Tailwind: A Better Solution Without Dependencies
TL;DR
Add this utility class to your globals.cc
file.
@layer utilities {
/* Chrome, Safari and Opera */
.scrollbar-hidden::-webkit-scrollbar {
display: none;
}
.scrollbar-hidden {
scrollbar-width: none; /* Firefox */
-ms-overflow-style: none; /* IE and Edge */
}
}
Context
I recently wrapped up Build UI's Tailwind Mastery course. Throughout the course we recreate Discords interface using pure Tailwind. In case you're curious, you can see the finish build here. While working through the course I encountered a common, but tricky styling challenge - scrollbars.
Discords UI requires scrollable sections for Servers, Channels, and Messages components. Given the narrow width of the Server and Channel sections, default scrollbars not only disrupt the clean design but actively detract from the user experience.
The instructor, Sam Selikoff, demonstrates how to accomplish this aesthetic using overflow-y-scroll
, but the default browser scrollbars clash hard with Discord's sleek design aesthetic.
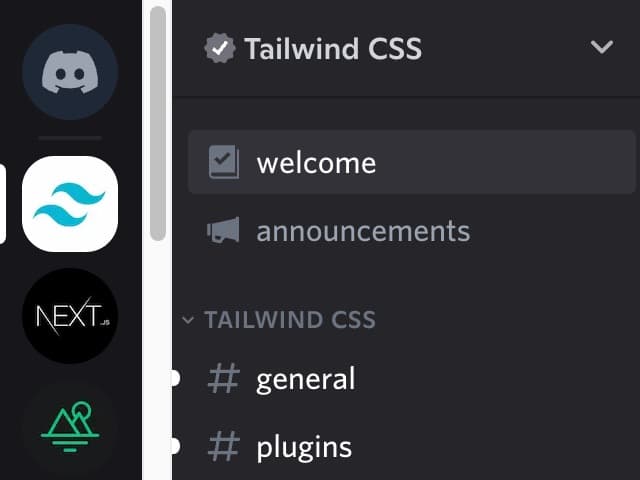
While overflow-y-scroll
provides the functionality, it falls short on the
design requirements.
The Quick Fix vs The Right Solution
Like many developers under time pressure, I initially reached for the quickest solution: the tailwind-scrollbar-hide plugin. A simple npm install
, add it to your Tailwind plugins, and you get access to scrollbar-hide
. Problem solved, right?
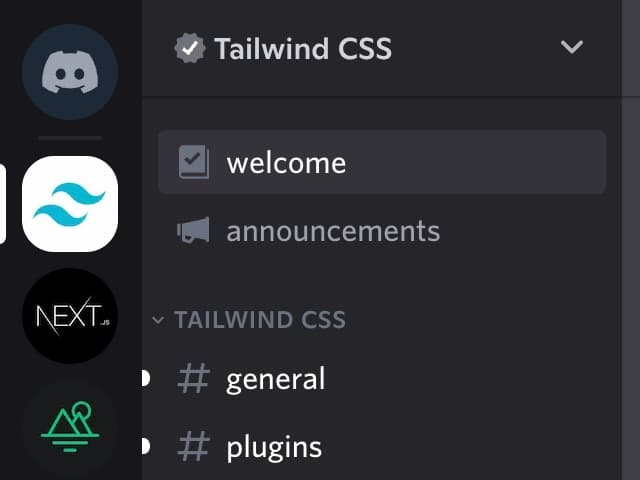
However, this approach introduces an unnecessary dependency for what turns out to be a straightforward CSS solution. While the plugin works, it violates one of the core principles of maintainable code: avoiding dependencies for problems that can be solved with a few lines of CSS.
A Better Approach
The real solution isn't about finding the right plugin—it's about adding our own custom style to Tailwind. While researching this, I found a great article by Subframe called Hide your scrollbars but still scroll with Tailwind. They showed how we can solve this with just a few lines of CSS, no extra packages required.
The Solution: Custom Utility Class
The key insight is that we don't need a plugin because Tailwind provides a built-in mechanism for adding custom utilities. While Tailwind doesn't include scrollbar styling out of the box, we can easily add it ourselves.
In your globals.css
file (using Tailwind v3.4.13), add this custom utility:
@layer utilities {
/* Chrome, Safari and Opera */
.scrollbar-hidden::-webkit-scrollbar {
display: none;
}
.scrollbar-hidden {
scrollbar-width: none; /* Firefox */
-ms-overflow-style: none; /* IE and Edge */
}
}
Don't worry if this looks complicated! It's doing something simple: creating a new class called scrollbar-hidden that you can use just like any other Tailwind class. The code looks complex because different web browsers need different instructions to hide scrollbars. Once you add this code, you can simply add scrollbar-hidden to any element where you want to hide the scrollbar while keeping the scrolling functionality.
Why This Matters
This solution offers several advantages:
- No additional dependencies to manage
- Zero impact on bundle size
- Full control over the implementation
- Better understanding of the underlying CSS
If you include this utility in your base Tailwind setup, it's available when needed but adds negligible overhead if unused. This makes it an ideal candidate for inclusion in your starter templates or design system.
Conclusion
This is a great example of a common situation in web development: sometimes installing a package seems like the quickest fix, but taking a moment to understand the problem often leads to a simpler solution. While plugins like tailwind-scrollbar-hide definitely have their place, creating our own simple utility class gives us a solution that's easier to maintain and helps us better understand how our code works. If you're curious about other ways to handle scrollbars in Tailwind, check out the complete Subframe article that inspired this solution.